BMeasure-lib 0.8.4 |
BMeasureApi::BMeasureUnits Class Reference
#include <BMeasureUnits.h>
Inheritance diagram for BMeasureApi::BMeasureUnits:
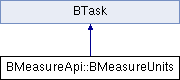
Public Member Functions | |
BMeasureUnits (Bool threaded=0) | |
virtual | ~BMeasureUnits () |
void | clear () |
BError | unitsFind () |
BError | unitAdd (BString serialNumber, BString device) |
BError | unitDelete (BString device) |
BUInt32 | unitsNum () |
BUInt32 | unitsConnectedNum () |
BMeasureUnit1 & | unit (BUInt u) |
BMeasureUnit1 & | unitMaster () |
BError | unitsConnect () |
Bool | unitsConnected () |
BError | unitsDisconnect () |
virtual void | disconnected () |
BError | unitSetOrder (BUInt u, BUInt order, Bool move) |
BError | unitSetEnabled (BUInt u, Bool enable) |
BError | dataSetNumStreams (BUInt num) |
Set the number of data output channels. More... | |
void | dataProcessEnable (Bool on) |
Enable the processing of data. More... | |
void | dataClear () |
BUInt | dataAvailable (BUInt stream) |
BError | dataWait (BUInt stream, BTimeout timeoutUs=BTimeoutForever) |
virtual void | dataEvent (BUInt stream) |
DataBlock * | dataRead (BUInt stream) |
void | dataDone (BUInt stream) |
void | run () |
Threaded run mode. More... | |
void | sendDataQueue (const DataBlock &dataBlock) |
void | sendDataProcess () |
void | sendDataProcessTrigger () |
void | outputBlock (BMeasureUnitsDataBlock *block) |
virtual BUInt | numChannels () |
The number of channels of data. More... | |
virtual BError | setMode (const Mode &mode) |
Set the current operational mode. More... | |
virtual BError | getStatus (NodeStatus &nodeStatus) |
virtual void | sendTime (const BTimeUs &time) |
Sends the current time. More... | |
virtual BError | getInformation (Information &info) |
virtual BError | getInfoBlock (InfoBlock &infoBlock) |
virtual BError | getChannelConfig (const BUInt8 &channelNumber, ChannelConfig &channelConfig) |
virtual BError | setChannelConfig (const BUInt8 &channelNumber, const ChannelConfig &channelConfig) |
virtual BError | getConfig (Configuration &config) |
Should we have this, not generic for different instruments ? More... | |
virtual BError | setConfig (const Configuration &config) |
Should we have this, not generic for different instruments ? More... | |
virtual BError | getMeasurementConfig (MeasurementConfig &measurement) |
Get measurement config. More... | |
virtual BError | setMeasurementConfig (const MeasurementConfig &measurement) |
Set measurement config. More... | |
virtual BError | getMeasurement (MeasurementConfig &measurement) |
Get measurement settings. More... | |
virtual BError | setMeasurement (const MeasurementConfig &measurement) |
Set measurement settings. More... | |
virtual BError | sendDataEnable (const DataSend &dataSend) |
Enables the sending of data. More... | |
virtual BError | getAwgConfig (AwgConfig &awgConfig) |
Get AWG Configuration. More... | |
virtual BError | setAwgConfig (const AwgConfig &awgConfig) |
Configure AWG. More... | |
virtual void | sendDataServe1 (const DataBlock &dataBlock) |
virtual void | sendMessage (BUInt32 &source, BString &message) |
virtual void | sendMessageServe (const BUInt32 &source, const BString &message) |
void | debugPrint () |
![]() | |
BTask (const char *name="", BUInt stackSize=0, BUInt priority=1) | |
~BTask () | |
void | init (const char *name, BUInt stackSize=0, BUInt priority=1) |
BError | start () |
void | stop () |
void | waitForCompletion () |
int | setPriority (BUInt priority) |
Private Member Functions | |
BMeasureUnitsDataBlock * | getFreeBlock (BUInt numSamples) |
Private Attributes | |
BSemaphoreBool | oprocEnable |
Enable processing. More... | |
BSemaphoreBool | oprocRunning |
Processing is running. More... | |
BMutex | olockUnits |
BList< BMeasureUnit1 * > | ounits |
BInt | ounitMaster |
BUInt | onumConnected |
BUInt | onumChannels |
BUInt | odataStreamNum |
BUInt32 | ofill |
BUInt | onumBlocks |
BMutex | olockInput |
BList< BMeasureUnitsDataBlock * > | odataBlocksFree |
BList< BMeasureUnitsDataBlock * > | odataBlocksIn |
BList< BMeasureUnitsDataBlock * > | odataBlocksProcess |
BCondInt | odataBlocksProcessNum |
BMutex | olockOutput |
BList< BMeasureUnitsDataBlock * > | odataBlocksOut [2] |
BCondInt | odataBlocksOutCount [2] |
MeasurementConfig | olocalTrigger |
Bool | otriggered |
BUInt | ostartSample |
Additional Inherited Members | |
![]() | |
static void * | taskFunc (void *) |
![]() | |
const char * | oname |
BUInt | ostackSize |
BUInt | opolicy |
BUInt | opriority |
pthread_t | othread |
Bool | orunning |
Constructor & Destructor Documentation
◆ BMeasureUnits()
BMeasureApi::BMeasureUnits::BMeasureUnits | ( | Bool | threaded = 0 | ) |
◆ ~BMeasureUnits()
| virtual |
Member Function Documentation
◆ clear()
void BMeasureApi::BMeasureUnits::clear | ( | ) |
◆ dataAvailable()
◆ dataClear()
void BMeasureApi::BMeasureUnits::dataClear | ( | ) |
◆ dataDone()
void BMeasureApi::BMeasureUnits::dataDone | ( | BUInt | stream | ) |
◆ dataEvent()
| virtual |
◆ dataProcessEnable()
void BMeasureApi::BMeasureUnits::dataProcessEnable | ( | Bool | on | ) |
Enable the processing of data.
◆ dataRead()
◆ dataSetNumStreams()
Set the number of data output channels.
◆ dataWait()
BError BMeasureApi::BMeasureUnits::dataWait | ( | BUInt | stream, |
BTimeout | timeoutUs = BTimeoutForever | ||
) |
◆ debugPrint()
void BMeasureApi::BMeasureUnits::debugPrint | ( | ) |
◆ disconnected()
| virtual |
◆ getAwgConfig()
Get AWG Configuration.
◆ getChannelConfig()
| virtual |
◆ getConfig()
| virtual |
Should we have this, not generic for different instruments ?
◆ getFreeBlock()
| private |
◆ getInfoBlock()
◆ getInformation()
| virtual |
◆ getMeasurement()
| virtual |
Get measurement settings.
◆ getMeasurementConfig()
| virtual |
Get measurement config.
◆ getStatus()
| virtual |
◆ numChannels()
| virtual |
The number of channels of data.
◆ outputBlock()
void BMeasureApi::BMeasureUnits::outputBlock | ( | BMeasureUnitsDataBlock * | block | ) |
◆ run()
| virtual |
Threaded run mode.
Reimplemented from BTask.
◆ sendDataEnable()
Enables the sending of data.
◆ sendDataProcess()
void BMeasureApi::BMeasureUnits::sendDataProcess | ( | ) |
◆ sendDataProcessTrigger()
void BMeasureApi::BMeasureUnits::sendDataProcessTrigger | ( | ) |
◆ sendDataQueue()
void BMeasureApi::BMeasureUnits::sendDataQueue | ( | const DataBlock & | dataBlock | ) |
◆ sendDataServe1()
| virtual |
◆ sendMessage()
◆ sendMessageServe()
| virtual |
◆ sendTime()
| virtual |
Sends the current time.
◆ setAwgConfig()
Configure AWG.
◆ setChannelConfig()
| virtual |
◆ setConfig()
| virtual |
Should we have this, not generic for different instruments ?
◆ setMeasurement()
| virtual |
Set measurement settings.
◆ setMeasurementConfig()
| virtual |
Set measurement config.
◆ setMode()
Set the current operational mode.
◆ unit()
BMeasureUnit1 & BMeasureApi::BMeasureUnits::unit | ( | BUInt | u | ) |
◆ unitAdd()
◆ unitDelete()
◆ unitMaster()
BMeasureUnit1 & BMeasureApi::BMeasureUnits::unitMaster | ( | ) |
◆ unitsConnect()
BError BMeasureApi::BMeasureUnits::unitsConnect | ( | ) |
◆ unitsConnected()
Bool BMeasureApi::BMeasureUnits::unitsConnected | ( | ) |
◆ unitsConnectedNum()
BUInt BMeasureApi::BMeasureUnits::unitsConnectedNum | ( | ) |
◆ unitsDisconnect()
BError BMeasureApi::BMeasureUnits::unitsDisconnect | ( | ) |
◆ unitSetEnabled()
◆ unitSetOrder()
◆ unitsFind()
BError BMeasureApi::BMeasureUnits::unitsFind | ( | ) |
◆ unitsNum()
BUInt BMeasureApi::BMeasureUnits::unitsNum | ( | ) |
Member Data Documentation
◆ odataBlocksFree
| private |
◆ odataBlocksIn
| private |
◆ odataBlocksOut
| private |
◆ odataBlocksOutCount
| private |
◆ odataBlocksProcess
| private |
◆ odataBlocksProcessNum
| private |
◆ odataStreamNum
| private |
◆ ofill
| private |
◆ olocalTrigger
| private |
◆ olockInput
| private |
◆ olockOutput
| private |
◆ olockUnits
| private |
◆ onumBlocks
| private |
◆ onumChannels
| private |
◆ onumConnected
| private |
◆ oprocEnable
| private |
Enable processing.
◆ oprocRunning
| private |
Processing is running.
◆ ostartSample
| private |
◆ otriggered
| private |
◆ ounitMaster
| private |
◆ ounits
| private |
The documentation for this class was generated from the following files:
Generated by
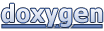